https://docs.python.org/ko/3/library/collections.html#counter-objects
collections — Container datatypes
Source code: Lib/collections/__init__.py This module implements specialized container datatypes providing alternatives to Python’s general purpose built-in containers, dict, list, set, and tuple.,,...
docs.python.org
Python의 collections 라이브러리에서 있는 Counter (계수기) 객체이다.
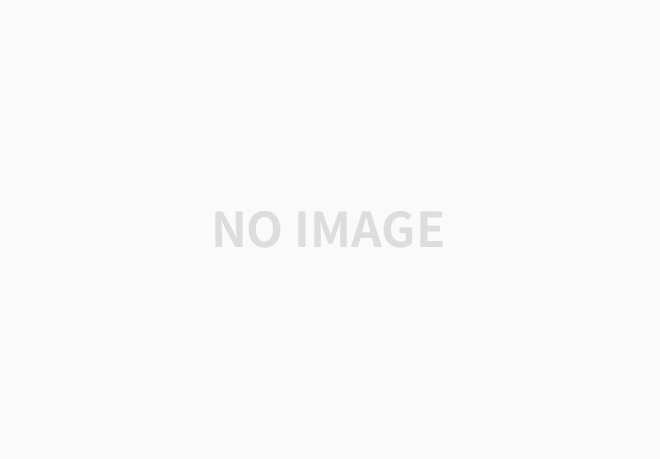
카운터는 해시가능한 객체들을 세는 딕셔너리의 서브클래스이다. 딕셔너리의 키와 그 키의 개수가 value로 저장된다. Counts는 0 이하인 값도 가능하다.
elements() 함수를 통해 카운터에 들어 있는 키들의 개수를 바탕으로, 리스트를 제작한다.
most_common(n) 함수를 통해 Counter에 들어 있는 n개의 제일 많은 것부터 적은 수대로 찾아준다.
total() 함수를 통해 총 개수를 알려준다.
collections에 있는 객체이므로, Collections에 있는 Counter를 import한다.
from collections import Counter
Counter를 초기화 할 수 있는 방법은 많다.
>>> c=Counter()
>>> c
Counter()
빈 Counter 객체이다.
>>> b=Counter({"HI": 3, "HELLO" : 4})
>>> b
Counter({'HELLO': 4, 'HI': 3})
string을 넣어서 초기화하였다.
>>> a=Counter("ABCDEFAB")
>>> a
Counter({'A': 2, 'B': 2, 'C': 1, 'D': 1, 'E': 1, 'F': 1})
Dictionary 객체가 들어갔다.
>>> a
Counter({'A': 2, 'B': 2, 'C': 1, 'D': 1, 'E': 1, 'F': 1})
>>> a["A"]
2
>>> a["Z"]
0
a라는 카운터가 존재할 때, a의 키를 딕셔너리처럼 구할 수 있다.
a 안에 "A"가 2개 있으므로, a["A"]는 2를 출력한다.
a 안에 "Z"는 없으므로, a["Z"]는 0을 출력한다.
일반적인 dictionary 객체에서는 key가 존재하지 않으면, KeyError을 출력한다.
>>> d={}
>>> d['A']=2
>>> d
{'A': 2}
>>> d["Z"]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'Z'
>>> a=Counter({'a':3, 'b':2, 'c':3})
>>> a['a']=0
>>> a
Counter({'c': 3, 'b': 2, 'a': 0})
>>> del a['b']
>>> a
Counter({'c': 3, 'a': 0})
>>>
a['a']를 통해 0으로 만들어줄 수 있다.
del을 쓰면, 아예 a가 Counter 내에 없어진다.
>>> d
Counter({'a': 6, 'f': 3, 's': 2, 'd': 2})
>>> d['s']-=10
>>> d
Counter({'a': 6, 'f': 3, 'd': 2, 's': -8})
>>> -d
Counter({'s': 8})
>>> +d
Counter({'a': 6, 'f': 3, 'd': 2})
Counter 내의 값은 음수도 가능하다.
-를 앞에 쓰면, Count가 음수인 것만 출력된다.
+를 앞에 쓰면, Count가 양수인 것만 출력된다.
>>> a=Counter("abc")
>>> b=Counter("aacc")
>>> a+b
Counter({'a': 3, 'c': 3, 'b': 1})
>>> a-b
Counter({'b': 1})
>>> a|b
Counter({'a': 2, 'c': 2, 'b': 1})
>>> a&b
Counter({'a': 1, 'c': 1})
>>> a==b
False
>>> a<=b
False
>>> c=Counter('a')
>>> a>=c
True
+를 통해 두 counter를 더한다. (합집합)
-를 통해 두 counter를 뺀다. (차집합)
|을 통해 값들 중에서 제일 큰 값을 가진다.
&을 통해 값들 중에서 제일 작은 값을 가진다.
==을 통해 두 Counter가 같은지, <=를 통해서 포함관계를 나타낸다.
'Computer Science > Python' 카테고리의 다른 글
[Python 3] BOJ 8983 사냥꾼 (0) | 2024.04.17 |
---|---|
Python: Replace 함수 (0) | 2024.03.10 |